Laravel's Contextual Attributes
Are you tired of constantly fetching the current user with request()->user()
, auth()->user()
or passing the Request
object to every controller method that needs it?
Laravel 11, with its continued support for PHP 8.1's attribute syntax, offers a more streamlined approach: by simply using the #[CurrentUser]
attribute, you can directly inject the authenticated user into your controller methods:
use App\Models\User;
use Illuminate\Container\Attributes\CurrentUser;
class AuthController
{
public function me(#[CurrentUser] User $user): User
{
return $user;
}
}
A Deeper Dive: Laravel Attributes
And if you didn't know, there's a whole world of other attributes waiting to be discovered! This blog post focused on contextual attributes, namely CurrentUser
, but for all the available PHP attributes you can use in your Laravel project, check out this blog post:
All Laravel PHP Attributes at Your Disposal
PHP attributes are a great way to add metadata to your classes, methods, and properties. Laravel provides a bunch of them out of the box, you can use in your applications.
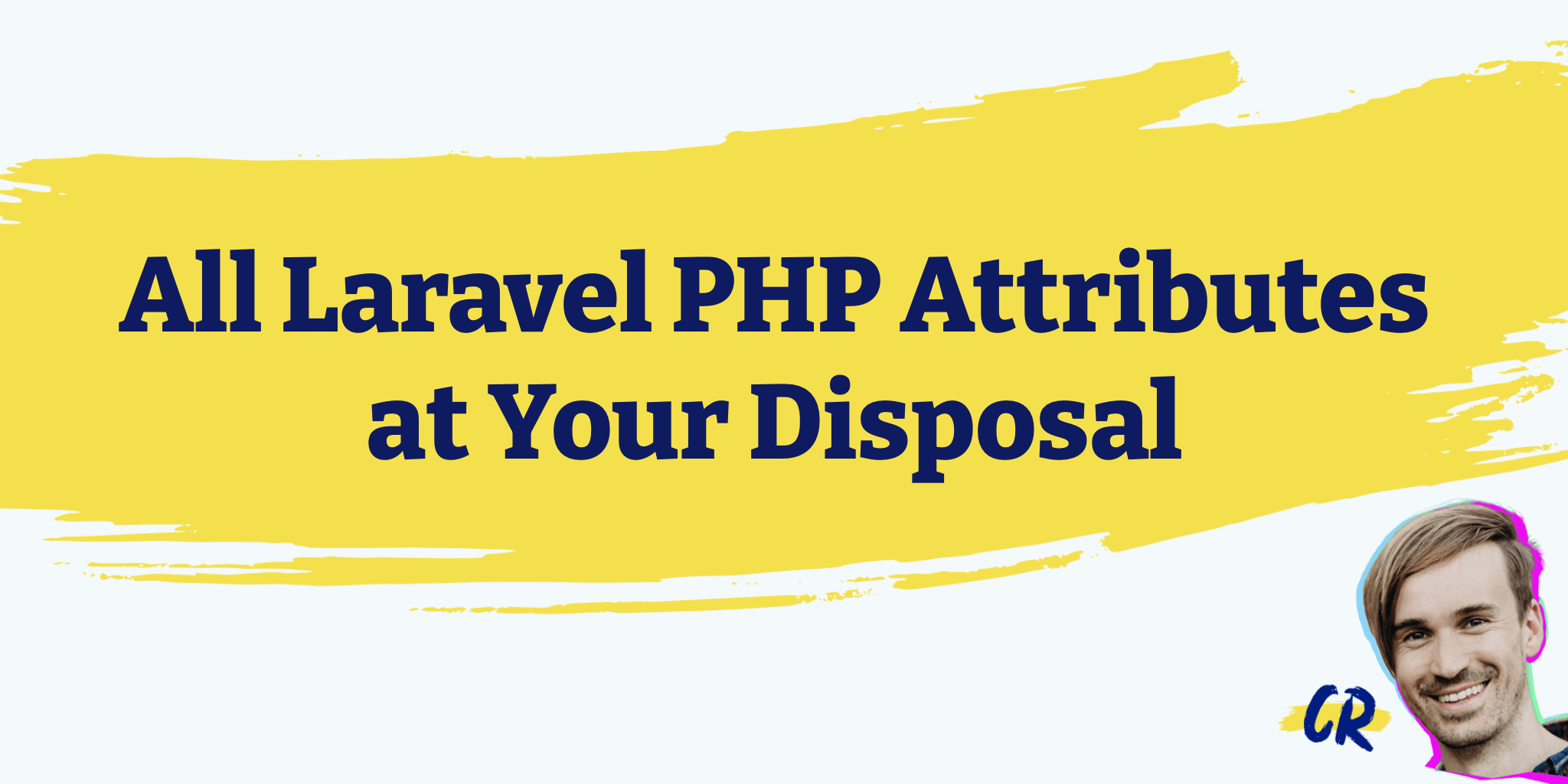
Member discussion